Google Analytics have different built-in integrations with other Google advertising platforms like Google Ads or Campaign Manager (in case of GA360) in order to import data from those platforms and present more complete reports. But in case of non-Google ad platforms like Facebook Ads – there are no built-in solutions within Google Analytics. It can be a problem if you want to analyze ROI (return of investment) of all your paid advertising channels and compare them. Of course you can build some custom reports outside of Google Analytics, but what if you want to do that analysis inside Google Analytics itself? I mean reports like this for example:

Then you should consider Google Analytics feature like Cost Data Import. That kind of import can be done manually by uploading csv file in Google Analytics panel, but in this tutorial I will show how to do it using Python and Google Analytics API – so you will be able to automate those kind of imports.
Create a Dataset in Google Analytics
First of all we need to create a dataset in Google Analytics itself. On this step we can determine what exactly kind of data we want to import into Google Analytics so it will tell us what columns does that data should have. You can create dataset in your Google Analytics Admin panel after clicking on “Data Import”:

Here just click “Create”:

Select “Cost Data” in Data Set type section:
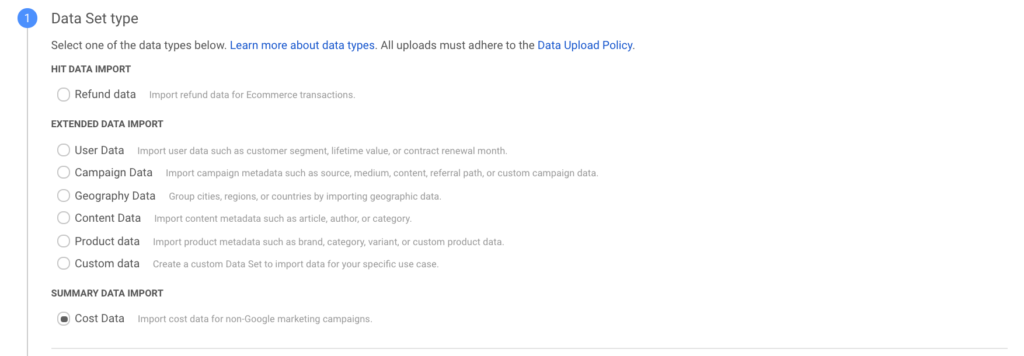
In “Data Set details” section – give your dataset a name and select in which views you would like to see those imported data:

The next step is very important because here You choose which metrics and dimensions you want to import and how data should behave in case of duplicates (for example you will import data for the same source and for the same day twice or more). I prefer “Overwrite” option but You should choose depending of your further script’s logic. So as a result You should have something like this in “Data Set schema” section:

Now click “Save”, then “Done” and that’s it – dataset has been created. That how it should look like now in Data Import section:

Click on dataset’s name and you will see two buttons: “Get Schema” and “Get Custom Data Source ID (for API users)”. Click them and save somewhere the values of both – you will need them later:
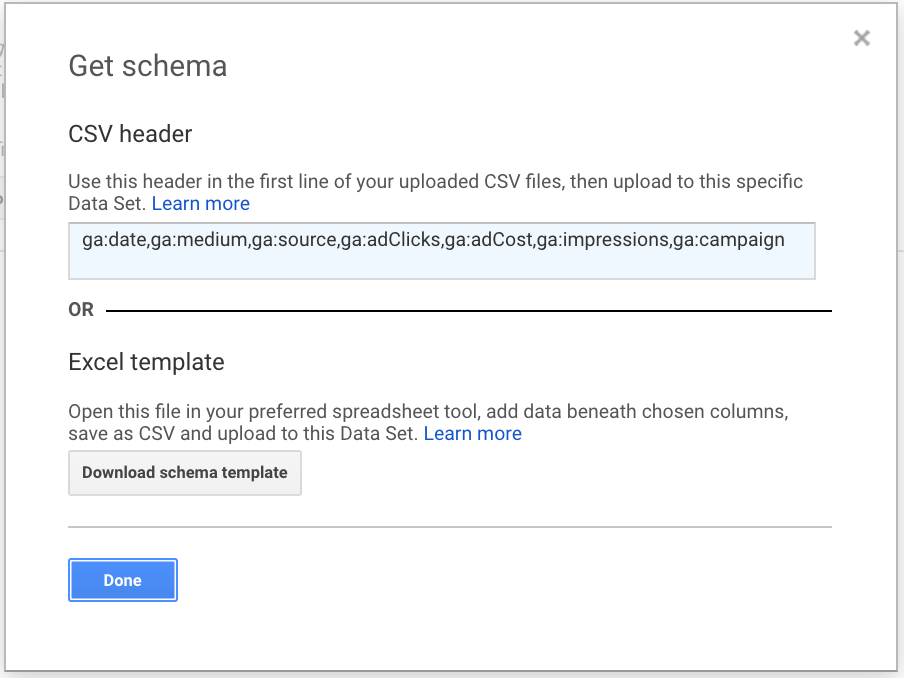

Send data using Google Analytics API and Python
Now we can try to send data into Google Analytics using Google Analytics Management API. I will not cover here basics of using Google Analytics API – you can read about it in my another article Google Analytics API Request (Python) and try it out. Here I will show you the code that will do one specific thing – it transforms a pandas dataframe to specific format so you will be able to send to Google Analytics. First of all – lets create a pandas dataframe with test data, that will contain well formatted values with proper column names:
import pandas as pd
df = pd.DataFrame({'ga:date': ['20201101'],
'ga:source': ['test_source'],
'ga:medium': ['test_medium'],
'ga:campaign': ['test_campaign'],
'ga:impressions': [10],
'ga:adClicks': [1],
'ga:adCost': [1.25],
})
I assume that you will extract those pandas dataframes from other advertising platforms like Facebook Ads using its APIs. For now we will try to upload our test dataframe that look like this:

As You’ve probably mentioned – our columns have the same names as schema of our dataset. It is important that the columns will have those names and proper data formats. Date column should have format %Y%m%d, so for example date 2020-11-01 will look like this: 20201101.
Next – make sure you have those python libraries installed in your project:
pip install google-api-python-client
pip install oauth2client
Now lets try to upload our test dataframe into Google Analytics:
import pandas as pd
import io
from googleapiclient.http import MediaIoBaseUpload
from google.oauth2 import service_account
from googleapiclient.discovery import build
df = pd.DataFrame({'ga:date': ['20201101'],
'ga:source': ['test_source'],
'ga:medium': ['test_medium'],
'ga:campaign': ['test_campaign'],
'ga:impressions': [10],
'ga:adClicks': [1],
'ga:adCost': [1.25],
})
# fill these variables with your values
KEY_FILE_LOCATION = 'your_service_account.json'
ACCOUNT_ID = 'account id'
PROPERTY_ID = 'property id'
DATASET_ID = 'dataset id'
###
credentials = service_account.Credentials.from_service_account_file(filename=KEY_FILE_LOCATION)
analytics = build('analytics', 'v3', credentials=credentials)
io_df = io.StringIO()
df.to_csv(io_df, index=False)
media = MediaIoBaseUpload(io_df, mimetype='application/octet-stream', resumable=False)
daily_upload = analytics.management().uploads().uploadData(
accountId=ACCOUNT_ID,
webPropertyId=PROPERTY_ID,
customDataSourceId=DATASET_ID,
media_body=media).execute()
Complete code above with your values and execute. If there are no errors – go to appropriate dataset in Google Analytics panel and check the status of upload:

If status is “Completed” – that means that it worked. If you see any errors -there – check them up and try to fix them. Now you cant go to “Cost Analysis” report in Google Analytics (Acquisition – Campaigns – Cost Analysis) and see if cost data are already there (it can take few minutes so the data will appear in reports):

If you will create those kind of script for all of your advertising channels using APIs and automate cost import into Google Analytics – you will be able to monitor ROI of your paid channels and will have cost data immediately in various Google Analytics reports. If it’s too complicated for you and you don’t know programming well – there are also some services that will do for you those king of costs imports into Google Analytics, for example popular OWOX BI or cheaper Pipelinica.
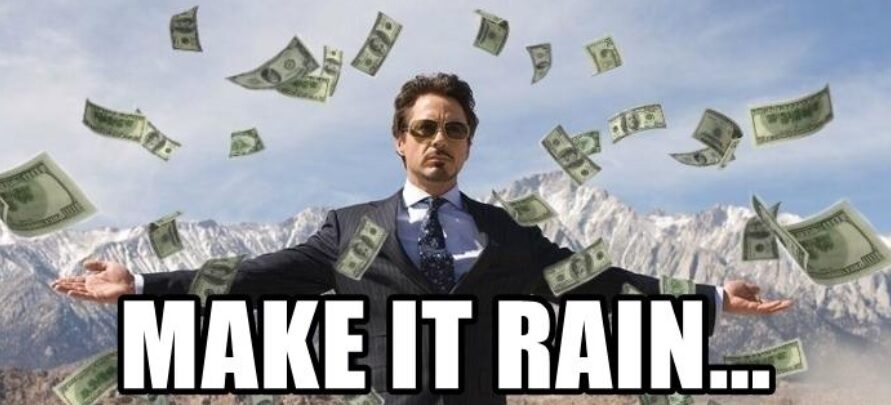